Arrays are useful critters because they can be used in many ways to store large amounts of data in a structured way. For example, a tic-tac-toe board can be held in an array. Arrays are essentially a way to store many values under the same name. You can make an array out of anys say you want to store astring, because C had no built-in datatype for strings, it was common to usearrays of characters to simulate strings. (C++ now has a string type as partof the standard library.)
For example: will allow you to declare a char array of 100 elements, or slots. Then you canreceive input into it from the user, and if the user types in a long string, it will go in the array. The neat thing is that it is very easy to work with strings in this way, and there is even a header file called cstring. There is another lesson on the uses of strings, so it's not necessary to discuss here.
Nov 14, 2019 In a C array declaration, the array size is specified after the variable name, not after the type name as in some other languages. The following example declares an array of 1000 doubles to be allocated on the stack.
The most useful aspect of arrays is multidimensional arrays. How I think about multi-dimensional arrays: This is a graphic of what a two-dimensional array looks like when I visualize it.
For example: declares an array that has two dimensions. Think of it as a chessboard. You can easily use this to store information about some kind of game or to write something like tic-tac-toe. To access it, all you need are two variables, one that goes in the first slot and one that goes in the second slot. You can even make a three dimensional array, though you probably won't need to. In fact, you could make a four-hundred dimensional array. It would be confusing to visualize, however. Arrays are treated like any other variable in most ways. You can modify one value in it by putting: or, for two dimensional arrays However, you should never attempt to write data past the last element of the array, such as when you have a 10 element array, and you try to write to the [10] element. The memory for the array that was allocated for it will only be ten locations in memory, but the next location could be anything, which could crash your computer.
You will find lots of useful things to do with arrays, from storing information about certain things under one name, to making games like tic-tac-toe. One suggestion I have is to use for loops when accessing arrays. Here you see that the loops work well because they increment the variable for you, and you onlyneed to increment by one. It's the easiest loop to read, and you access the entire array.
One thing that arrays don't require that other variables do, is a reference operator whenyou want to have a pointer to the string. For example: As opposed to The reason for this is that when an array name is used as an expression, it refers to a pointer to the first element, not the entire array. This rule causes a great deal of confusion, for more information please see our Frequently Asked Questions.
Quiz yourself
Previous: Structures
Next: Strings
Back to C++ Tutorial Index
C Programming Arrays and strings |
Arrays in C act to store related data under a single variable name with an index, also known as a subscript. It is easiest to think of an array as simply a list or ordered grouping for variables of the same type. As such, arrays often help a programmer organize collections of data efficiently and intuitively.
Later we will consider the concept of a pointer, fundamental to C, which extends the nature of the array (array can be termed as a constant pointer). For now, we will consider just their declaration and their use.
Arrays[edit]
C arrays are declared in the following form:
For example, if we want an array of six integers (or whole numbers), we write in C:
For a six character array called letters,
and so on.
You can also initialize as you declare. Just put the initial elements in curly brackets separated by commas as the initial value:
For example, if we want to initialize an array with six integers, with 0, 0, 1, 0, 0, 0 as the initial values:
Though when the array is initialized as in this case, the array dimension may be omitted, and the array will be automatically sized to hold the initial data:
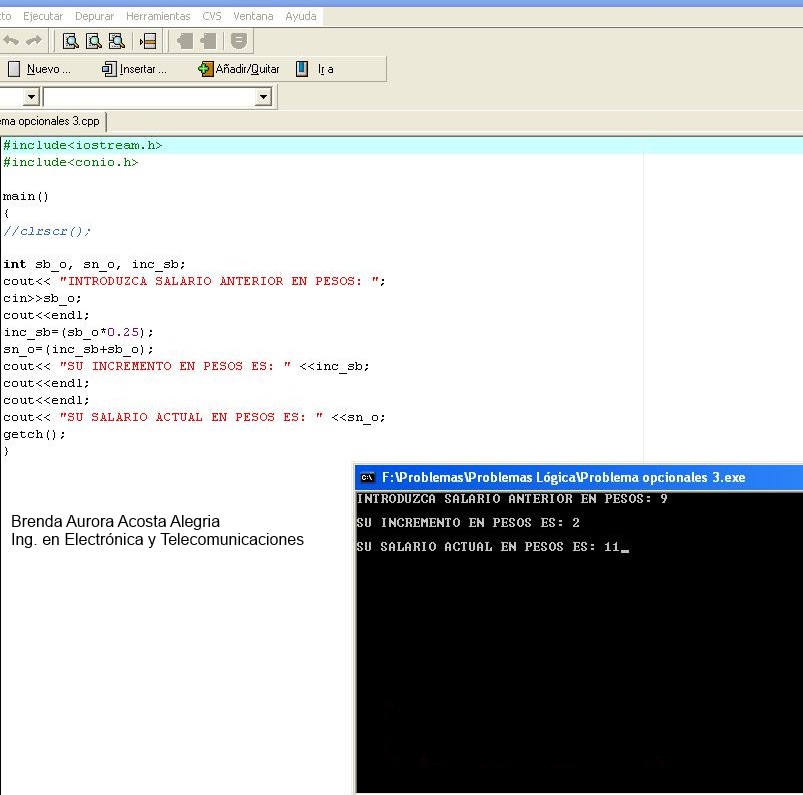
This is very useful in that the size of the array can be controlled by simply adding or removing initializer elements from the definition without the need to adjust the dimension.
If the dimension is specified, but not all elements in the array are initialized, the remaining elements will contain a value of 0. This is very useful, especially when we have very large arrays.
The above example sets the first value of the array to 245, and the rest to 0.
Dev C++ For Windows 10
If we want to access a variable stored in an array, for example with the above declaration, the following code will store a 1 in the variable x
Arrays in C are indexed starting at 0, as opposed to starting at 1. The first element of the array above is point[0]. The index to the last value in the array is the array size minus one.In the example above the subscripts run from 0 through 5. C does not guarantee bounds checking on array accesses. The compiler may not complain about the following (though the best compilers do):
During program execution, an out of bounds array access does not always cause a run time error. Your program may happily continue after retrieving a value from point[-1]. To alleviate indexing problems, the sizeof() expression is commonly used when coding loops that process arrays.
Many people use a macro that in turn uses sizeof() to find the number of elements in an array,a macro variously named'lengthof()',[1]'MY_ARRAY_SIZE()' or 'NUM_ELEM()',[2]'SIZEOF_STATIC_ARRAY()',[3]etc.
Notice in the above example, the size of the array was not explicitly specified. The compiler knows to size it at 5 because of the five values in the initializer list. Adding an additional value to the list will cause it to be sized to six, and because of the sizeof expression in the for loop, the code automatically adjusts to this change. Good programming practice is to declare a variable size , and store the number of elements in the array in it.
size = sizeof(anArray)/sizeof(short)
C also supports multi dimensional arrays (or, rather, arrays of arrays). The simplest type is a two dimensional array. This creates a rectangular array - each row has the same number of columns. To get a char array with 3 rows and 5 columns we write in C
To access/modify a value in this array we need two subscripts:
or
Similarly, a multi-dimensional array can be initialized like this:
The amount of columns must be explicitly stated; however, the compiler will find the appropriate amount of rows based on the initializer list.
There are also weird notations possible:
a[i] and i[a] refer to the same location. (This is explained later in the next Chapter.)
Strings[edit]
C has no string handling facilities built in; consequently, strings are defined as arrays of characters. C allows a character array to be represented by a character string rather than a list of characters, with the null terminating character automatically added to the end. For example, to store the string 'Merkkijono', we would write
or
In the first example, the string will have a null character automatically appended to the end by the compiler; by convention, library functions expect strings to be terminated by a null character. The latter declaration indicates individual elements, and as such the null terminator needs to be added manually.
Strings do not always have to be linked to an explicit variable. As you have seen already, a string of characters can be created directly as an unnamed string that is used directly (as with the printf functions.)
C++ Array List
To create an extra long string, you will have to split the string into multiple sections, by closing the first section with a quote, and recommencing the string on the next line (also starting and ending in a quote):
While strings may also span multiple lines by putting the backslash character at the end of the line, this method is deprecated.
There is a useful library of string handling routines which you can use by including another header file.
Arrays En Dev C Ing En Dev C++ Con Get
This standard string library will allow various tasks to be performed on strings, and is discussed in the Strings chapter.
References[edit]
- ↑Pádraig Brady.'C and C++ notes'.
- ↑C Programming/Pointers and arrays
- ↑MINC/Reference/MINC1-volumeio-programmers-reference
Using Arrays In Dev C++
Dev C++ 5.11

C Programming Arrays and strings |